Locations are complete!
Rooms are looking nice.
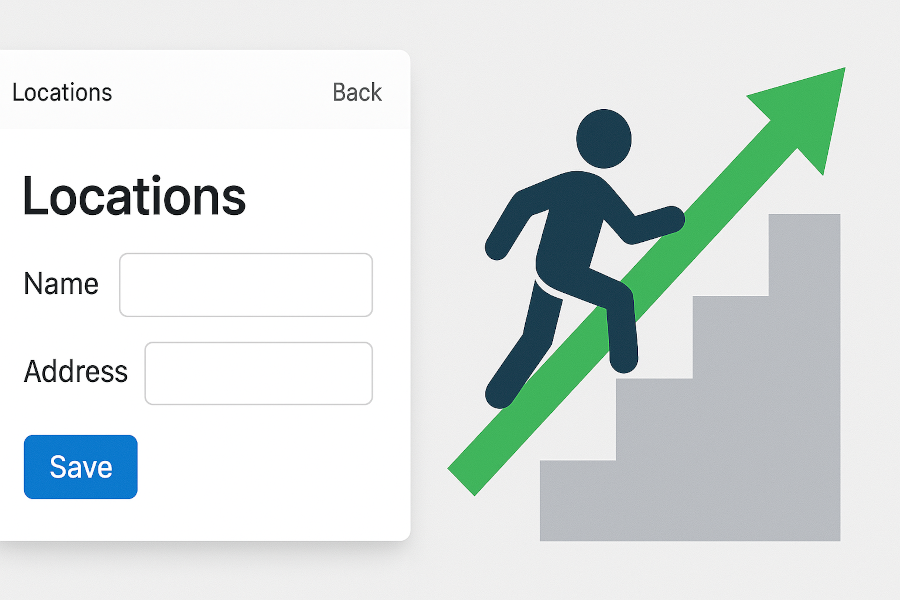
This is pretty large update with a really neat feature that I really liked coding. Let's get to the updates.
Create Campuses and allow the editing of them
Done. I created this first, but finished it last. When it came time for campuses, I decided to go all out on the prettiness angle (I'm not good at it, but I think it came out pretty good). I added pictured to display of the campus, as well as made those pictures overlays in certain cases. I also am able to attach an SVG icon and color it to the users preferences. I plan to use that icon in locations where putting in the campuses' full name is too much.
When I was creating the campuses, I wanted to have a place to show the actual campus. When I created the "how" route, I decided to copy the style of the personal profile. This means that the style I got from the template site that I've been using as a personal profile can be adapted to the show the “profile” of the campuses. It came out really good, allowing me to copy the layout from the person edit as well. What I have now is a pretty seamless experience that looks similar across pages.
Along with these upgrades, I enhanced the address and phone system. Now I can give a model the traits Addressable or Phoneneable to have them be able to link to multiple addresses or phones. Alternatively, the traits SingleAddressable and SinglePhoneable will let the system know to only attach a single phone number/address to the model. Because of this, I can now add phones number and addresses to almost any model. So Campuses can have multiple addresses and multiple phones attached.
Create Years and allow the editing of them
Done. Years turned out pretty easy. I combined them with terms below to create a single interface for them.
Create Semesters and allow the editing of them
Done. Terms are done through a Livewire Widgets, that allows me to define multiple terms in a single year for multiple campuses. The look like this:
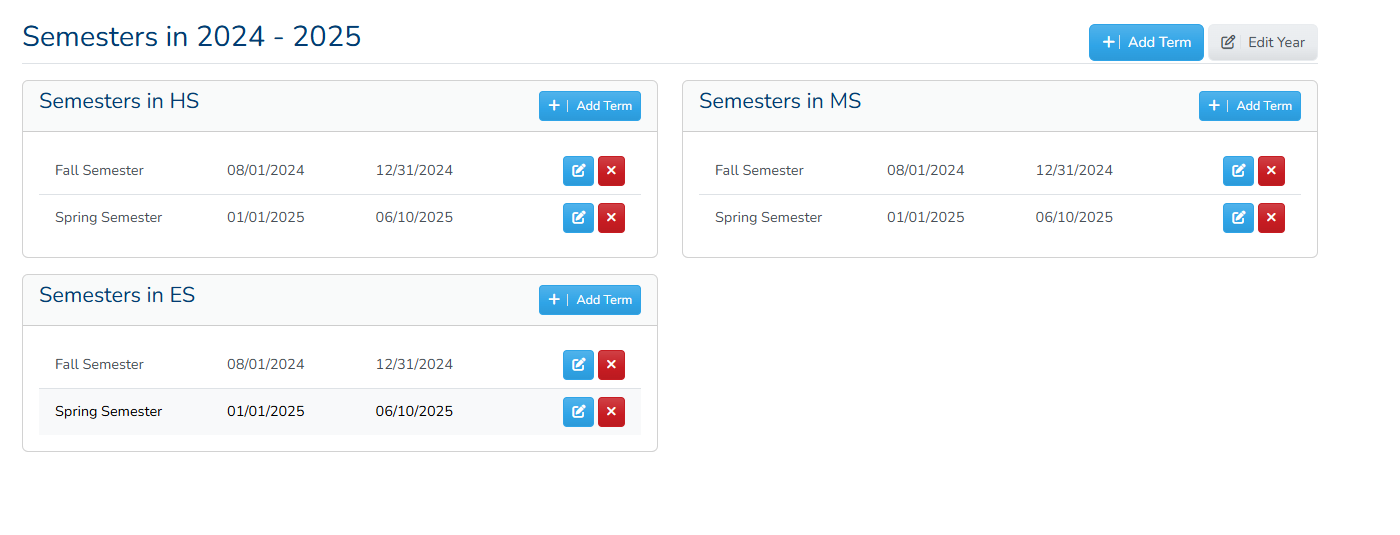
Create Rooms and the structures around them to begin creating a school
This was the piece that took me the longest. I would have released this 2 weeks ago if it wasn't for this piece. Originally, I was simply going to create rooms and have them be attached to the campus. After more thinking, I realized that this would be the place to build the campus maps. One of the things every School needs is a Campus Map. These maps are given to students and visitors so that they can find their way on campus. These maps usually need to be updated pretty regularly to update the classroom names or class being taught. I decided that I wanted the Campus Map to be an integral part of the system by allowing users to build these maps through the Rooms system.
First lets take a look at the updated class diagram:
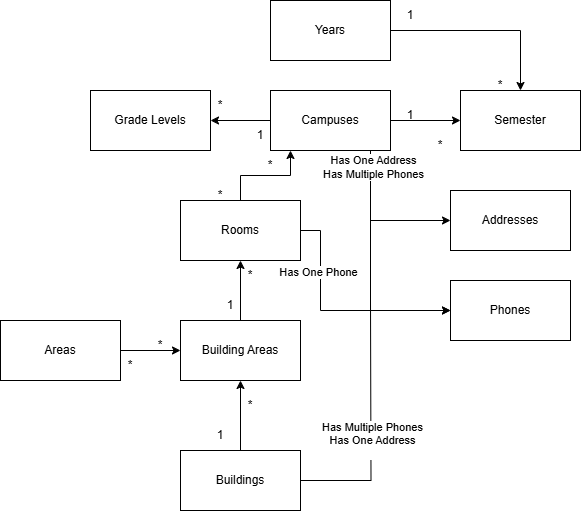
We have a new Table called “Areas”. This is a CRUD table that keeps the names of the areas for the building to use, such as 1st Floor, 2nd Floor, etc. The Building table now holds all the Buildings in the system. These are different than campuses, which can contain multiple buildings. The Building table is pretty simple, only really containing the image that is assigned to the campus, and links to a single address (building can only physically be in a single address) and multiple phones. The combination of Areas and Building leads to a new table Building Areas, which is the container that describes a single area in a building where rooms are located. This container holds a very important link, which is the URL to the floor blueprint.
Now, every Room belongs to a BuildingArea, which means that the physical location of that Room is in that Building/Area combination. Since we also have an image of the blueprints, we can now “draw” on the blueprint the actual location of the Room. I made this into a JS module called AreaDrawing, and a collection of all AreaDrawings in a BuildingArea is now bundled into a MapDrawings. Thus, I can define the bounds of a room, store that data into the Rooms table, and then I can show an interactive map of that floor, with all the rooms highlighted and hoverable, and can even tie in an action when the user clicks on a room.
The whole thing is not tight as I want it yet. I would like the editor and map to be able to switch floors without having to rely on external links. I would also like to make sure the campuses are easy to navigate. I will tackle this later, as I wanted to continue building the rest, else I could spend months in this area alone, which I can always do later when I have a more finished system.
Seed all these new components.
Done! I reworked the system so that there are two buildings: a 2-story building and a 3-story one, each one has blueprints on each floor and I even defined all the rooms in the 2-story building, and 12 rooms in the 3-story one. I also tied the HS campus to the 2-story building, the MS campus to the 3-story building and the ES campus to both the 2-story and 3-story buildings. I will eventually like to define all areas in the map, but it is overkill for now, and it was getting tedious.
The school looks a bit more put together after this update, having the second part of the trinity partially built.
Subject Matter and Interactions
So looking back at our original diagram:
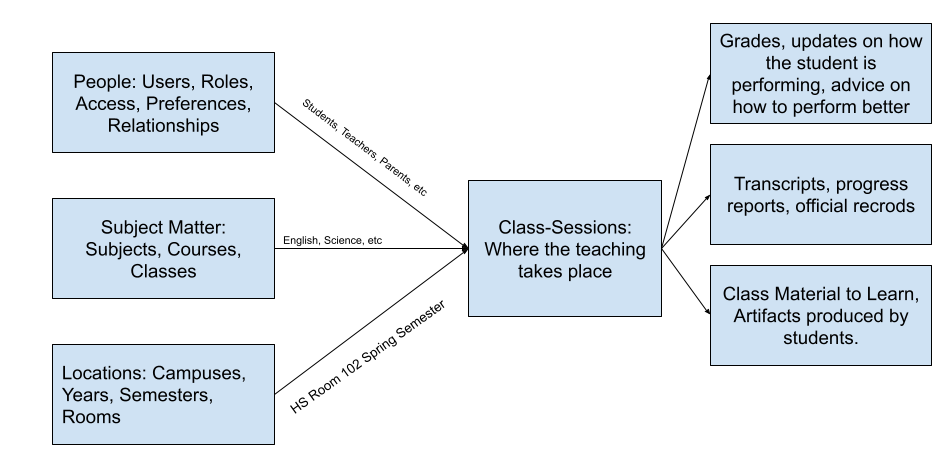
We see that we have People and Locations partially built, so we need to build Subject Matter next. While that is true, there are interactions between the 3 major components listed here that are not really defined in this diagram. So there will be interactions between the People and Locations, between People and Subject Matter, and Subject Matter and Locations. Since we don't really have Subject Matter built, we need to concentrate on the People-Locations interactions, which have two major interactions (and a few minor ones as well): Campus and People for campuses the people belong to and Campus, Person and Year for the Student Record.
Campus-Person
One of the most important interactions between the People and Locations system is the personal campus (or campuses) for a Person. The connections are different though, depending on the kind or Person record accessing the campuses. Virtually every Person will be connected to a Campus in some capacity, only each will be done in different ways.
Employees will be linked to one or multiple campuses based on where they work. An employee can be assigned to work at one or multiple campuses in different capacities. Faculty will teach at certain campuses, which Staff would administer certain things in one or more campuses as well. For example we can have a teacher that teaches Math in both the HS and MS, or a School Registrar, that handles transcript requests for all campuses, or a campus secretary, that handles requests for a single campus. This kind of connection is called an EmployeeCampus connection, which could be further specified into a TeachingCampus connection for teachers, and a StaffCampus connection for staff. But is it worth having it that fine-grained?
Students are linked to a single Campus via the StudentRecord. The student record is a very special relationship that I will describe in the next section, but suffice it to say that Students can only be linked to a single campus at any one time.
Parents are linked to one or more Campuses through their students. Parents are not really "directly" linked to campuses, rather they are indirectly linked through whatever student or students they are related to. The role Parent is only assigned to a Person that has a child-parent relationship and where the child person is also a student. Since the Student record is tied to a Campus, parents get their campus relationship depending on where their students are currently attending. A parent with a single student in the HS will have the HS campus assigned, a parent with a student in the MS and the ES, will have those two campuses attached. The big question is whether it is worth directly linking them, or to use the relationship through the student record. A direct link will make it easier to access, but will need to be updated every year and every time a relationship is changes, while an indirect link will take more queries but will not need to be updated.
The Student Record
This is the hardest record to deal with, but the most key. A StudentRecord connection is assigned to a Person-Campus-Year relationship that designates that the Person is attending the Campus for the Year. This is always time sensitive when dealing with the CurrentYear, but also important when looking at a student's progress throughout the years at the school.
It is expected for a Person record that has either the Student or Old Student role to at least one StudentRecord, and having more likely more than one. This record will also be linked to the Levels CRUD table, since it designates which grade they're currently coursing. The table structure of the StudentRecord will look something like this:
Field | Note |
---|---|
id | The id of the record. It will have it's own id so we can link other things to it, such as classes, etc. |
campus | The Campus that this student is attending |
year | The Year that this record is for |
person | The Person this record refers to |
level | The "grade" (or year, or whatever) the person is in during this year in this campus |
start | When the student started this year. Mainly useful for students who start the year late. Most students will just have this set to the start of the year date. |
end | Slightly different, this will stay null if the student completed the year, and will have a date if the student withdrew early for some reason. |
dismissal_type | A link to a CRUD table that will specify all the reasons for the student to have left in the middle of the year, such as expulsion, withdrew, emergency, etc. |
dismissal_note | Optional note of why the student was dismissed. |
note | Private note about the student. Only shown to people with the correct permission. |
active | Whether this is the current active record for this Person. So we can easily look up the current StudentRecord for a Person. |
time stamps | The normal timestamps. |
Putting it all together
So now we have all the major interactions, we can develop a diagram of what it looks like. The details are sketchy and subject to change, but I'll be more detailed when I get them finalized.
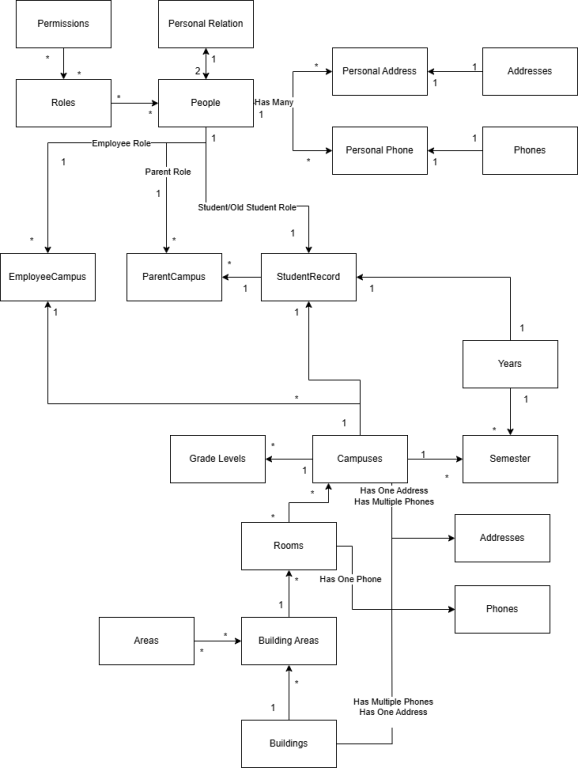
But wait! There's more!
Subject Matter is special in the sense that it really can't be built without the Locations piece, as it relies heavily on it. In fact the most basic Subject Matter model, aptly named SubjectMatter, has a direct link to Campus, as it is the subject matter that is taught in a campus. So since we have the locations piece in place and we're currently dealing with interactions, it makes sense to put in at least the basics of the Subject Matter system: SubjectMatters and Courses.
SubjectMatter is essentially a subject being taught at a campus. This is the most top-level you can get in terms of what is being taught at school. It should include things like “Mathematics” or “Science”, which avoiding being too descriptive, but not things like “Creative Writing”, since that might fall under “English”, or maybe “Language Arts”. In certain schools, they like to refer to this as Departments, since the “English Department” is usually in charge of all English-related courses, such as Creative Writing, or 9th Grade English. I don't like this idea, simply because a lot of the times departments handle more than one subject matter, such as the Athletics Department also doing Health, or the English department also doing Theater. So I refer to this as simple subject matter, that will be related to a Campus where it being taught, and will be refined further.
Courses is the refinement of SubjectMatter, specifying what material is taught. Where as the subject matter might be "Math", the Courses container would be things like "Algebra", "Algebra 2", "Calculus" in the HS Campus, or "7th Grade Math" in the MS Campus. A Course is basically the name of the class at contains all the materials that a class should be using, but it is not tied to a Year because it is not temporal. So this container will be used to link things like Scope and Sequence (more on that way later), or rubrics, or anything else related to the content of the class, but not the actual record of the class, which will be something else entirely.
Making these models will have the foundation of the Subject Matter System built, which we can use to define the rest of the interactions between the three systems as we head closer to the ClassSession model that will bring everything together.
With all that in mind here are the goals for the next release:
- Create the Student Record
- Create the interaction between Employees and Campuses
- Create the interaction between Parents and Campuses
- Create Subject Matter and allow the editing of it.
- Create the Courses and allow the editing of it.
I will no longer be doing update on DiCMS, as it will be handled in its own blog. Wil update when done.